PHP Gallery
1 post
Page 1 of 1
Ok it's little bit more advanced simple gallery.
So.. Let's start:
First we must create a new database and upload these tables.
config.php
index.php
![Image]()
![Image]()
![Image]()
I hope you like it
And I hope it helped someone
So.. Let's start:
First we must create a new database and upload these tables.
Code: Select all
Now it's time for config.phpCREATE TABLE IF NOT EXISTS `album` (
`id` int(15) NOT NULL auto_increment,
`name` varchar(100) NOT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=latin1 AUTO_INCREMENT=1 ;
CREATE TABLE IF NOT EXISTS `images` (
`pic_id` int(15) NOT NULL auto_increment,
`album_id` varchar(100) NOT NULL,
`real_name` varchar(100) NOT NULL,
`name` varchar(100) NOT NULL,
`size` varchar(50) NOT NULL,
`date` varchar(50) NOT NULL,
PRIMARY KEY (`pic_id`)
) ENGINE=InnoDB DEFAULT CHARSET=latin1 AUTO_INCREMENT=1 ;
config.php
Code: Select all
Now when it's done.. It's time for index.php<?php
// Let's connect to our database
$mysql_con = mysql_connect("localhost", "usernameHere", "passwordHere");
// If there are any problems, display an error
if(!$mysql_con){
die("Can not connect to database: <b><i>".mysql_error()."</i></b>");
}
// Select our database
$mysql_select_db = mysql_select_db("databaseNameHere", $mysql_con);
// If there's something wrong, display an error
if(!$mysql_select_db){
die("Can not select database: <b><i>".mysql_error()."</i></b>");
}
?>
index.php
Code: Select all
and here's thumb.php
<?php
include("config.php");
// Let's start our class
class album{
// Form for creating a new album
function create_new_album_form(){
echo "<b>Create new album</b><br /><br />";
echo "<form action='index.php?act=new&do=create' method='post'>";
echo "Album name:<br /><input type='text' name='album_name' /><br /><input type='submit' value='Create' />";
echo "</form>";
}
// Here we create a new album
function create_new_album(){
// Albums new name
$album_name = addslashes(htmlentities(htmlspecialchars($_REQUEST['album_name'])));
// If there's nothing entered, display an error
if($album_name == ""){
die("Please enter your album's name!");
}
$sql = "SELECT * FROM album WHERE name='".mysql_real_escape_string($album_name)."'";
$query = mysql_query($sql);
// Check is there any albums already named like this
if(mysql_num_rows($query)>0){
// If this name is already in use, display an error
die("This name is already in use! Please choose another name.");
}else{
// If this name is not in use, add it to database
$sql = "INSERT INTO album (name) VALUES ('".$album_name."')";
$query = mysql_query($sql);
if(!$query){
die("Can not create a new album: <b><i>".mysql_error()."</i></b>");
}else{
$sql = "SELECT * FROM album WHERE name='".mysql_real_escape_string($album_name)."'";
$query = mysql_query($sql);
if(!$query){
die(mysql_error());
}else{
$row = mysql_fetch_array($query);
$album_id = $row['id'];
}
// If album was successfully created, display message
echo "Album created! <a href='index.php?act=view&id=".$album_id."'>View</a>";
}
}
}
// Form for uploading your file
function upload_image_form(){
// Let's create a unique name for your image
$date = date("YmdHis");
$micro = explode(".", microtime(true));
$uniname = $date."".$micro['1'];
$album_id = addslashes(htmlentities(htmlspecialchars($_REQUEST['id'])));
// If album's id is wrong, display an error
if(!is_numeric($album_id)){
die("Wrong album ID!");
}else{
// Display file uploading form
echo "Upload new image<br /><br />";
echo "<i>You can upload: JPG, GIF and PNG images.</i><br />";
echo "<form enctype='multipart/form-data' action='index.php?act=upload&do=add&id=".$album_id."' method='post'>";
echo "Select file: <br />";
echo "<input type='file' name='file' /><br />";
echo "<input type='hidden' name='new_name' value='".$uniname."' />";
echo "<input type='submit' value='Upload' />";
echo "</form>";
}
}
// This function uploads your image
function upload_image(){
// Let's get all info that we need for uploading our image
$file_name = $_FILES['file']['name'];
$file_size = $_FILES['file']['size'];
$file_type = $_FILES['file']['type'];
$file_tmp = $_FILES['file']['tmp_name'];
$max_size = "512000"; # You can change this number. at the moment max file size is 500kb #
$file_ext_exp = explode(".", $file_name);
$file_ext = $file_ext_exp['1'];
$uni = addslashes(htmlentities(htmlspecialchars($_REQUEST['new_name'])));
$new_name = $uni.".".$file_ext;
$date = date("Y-m-d H:i:s");
$album_id = addslashes(htmlentities(htmlspecialchars($_REQUEST['id'])));
// If a file wasn't selected, display an error
if($file_name == ""){
die("Please select a picture!");
}else{
// Let's specify what image types we allow to upload
$types = array("image/jpg", "image/jpeg", "image/gif" , "image/png");
// If an user uploads different type of image, display an error
if(!in_array($file_type, $types)){
die("You can not upload this type of files! <a href='javascript:history.go(-1);'>Go back!</a>");
}else{
// If type is OK but image size is too big, display an error
if($file_size>$max_size){
die("Your image is too big! Max image size is: <b>500kb</b> <a href='javascript:history.go(-1);'>Select another file</a>");
}else{
// If everything is good, let's upload our file
$move = move_uploaded_file($file_tmp, "http://forum.codecall.net/images/".$new_name);
// If there was something wrong with uploading file, display an error
// The problem might be that your 'images' folder don't have correct permissions
// Change 'images' folder's CHMOD to 0777 or just 777
if(!$move){
die("Can not upload files to album!");
}else{
// If our file has been uploaded let's add it's info to database
$sql = "INSERT INTO images (album_id, name, size, date, real_name) VALUES ('".$album_id."', '".$new_name."', '".$file_size."', '".$date."', '".$file_name."')";
$query = mysql_query($sql);
// If there is something wrong with adding info to database, display an error
if(!$query){
die("Can not insert data to database: <b>".mysql_error()."</b>");
}else{
// Image is now uploaded, display a link to it
echo "Image is uploaded. <a href='index.php?act=view&id=".$album_id."'>View album</a>";
}
}
}
}
}
}
// This shows you your album
function view_album(){
// Let's get album's id
$album_id = addslashes(htmlentities(htmlspecialchars($_REQUEST['id'])));
$sql = "SELECT * FROM album WHERE id='".mysql_real_escape_string($album_id)."'";
$query = mysql_query($sql);
// If there aren't any records of this id, display an error
if(!$query){
die("Wrong ID!");
}else{
$row = mysql_fetch_array($query);
// Let's find all images that are in our album
$sql = "SELECT * FROM images WHERE album_id='".mysql_real_escape_string($album_id)."'";
$query = mysql_query($sql);
// If there aren't any images, display a message
if(mysql_num_rows($query)<1){
echo "Your album is empty! <a href='index.php?act=upload&id=".$album_id."'>Upload image</a>";
}else{
// If there are records of images, let's display them
echo "<a href='index.php'>Home</a> » <a href='index.php?act=view&id=".$album_id."'>".$row['name']."</a><br /><br />";
echo "<a href='index.php?act=upload&id=".$album_id."'>Upload image</a><br /><br />";
echo "<table border='0'>";
echo "<tr>";
$picnum = "1";
// Let's display our images as thumbnails
while($row = mysql_fetch_array($query)){
echo "<td>";
echo "<table border='0'>";
echo "<tr>";
echo "<td align='center'>";
echo "<a href='index.php?viewpic=".$picnum."&aid=".$album_id."'><img src='thumb.php?id=".$row['pic_id']."' border='0' /></a>";
echo "</td>";
echo "</tr>";
echo "<tr>";
echo "<td align='center'>";
echo "<a href='index.php?viewpic=".$picnum."&aid=".$album_id."'>".$row['real_name']."</a>";
echo "</td>";
echo "</tr>";
echo "</table>";
echo "</td>";
$picnum++;
}
echo "</tr>";
echo "</table>";
}
}
}
// Displays list of created albums
function list_albums(){
// Let's get records of our albums and list them
$sql = "SELECT * FROM album";
$query = mysql_query($sql);
if(mysql_num_rows($query)>0){
if(!$query){
die(mysql_error());
}else{
while($row = mysql_fetch_array($query)){
echo "<a href='index.php?act=view&id=".$row['id']."'>".$row['name']."</a> ";
}
}
}else{
// If there aren't any albums created, display a message
echo "There are no albums created. <a href='index.php?act=new'>Create album</a>";
}
}
// This displays your uploaded images
function view_picture(){
$album_id = addslashes(htmlentities(htmlspecialchars($_REQUEST['aid'])));
$pic = addslashes(htmlentities(htmlspecialchars($_REQUEST['viewpic'])));
// Let's get all images from our album
$sql = "SELECT * FROM images WHERE album_id='".mysql_real_escape_string($album_id)."'";
$query = mysql_query($sql);
$num_rows = mysql_num_rows($query);
// Pagination start
// Display links 'Next »' and « Previous links for naviation
$pic_page = "1";
$last_page = ceil($num_rows/$pic_page);
if(isset($pic)){
$picid = $pic;
}else{
$picid = "1";
}
if($picid>$last_page){
$picid = $last_page;
}
if($picid<1){
$picid = "1";
}
$sql = "SELECT * FROM album WHERE id='".mysql_real_escape_string($album_id)."'";
$query = mysql_query($sql);
if(!$query){
die("Wrong ID!");
}
$row = mysql_fetch_array($query);
echo "<a href='index.php'>Home</a> » <a href='index.php?act=view&id=".$album_id."'>".$row['name']."</a><br /><br />";
$limit = "LIMIT ".($picid-1)/$pic_page.','.$pic_page;
$sql = "SELECT * FROM images WHERE album_id='".mysql_real_escape_string($album_id)."' ".$limit;
$query = mysql_query($sql);
$row = mysql_fetch_array($query);
$image = "http://forum.codecall.net/images/".$row['name'];
if($picid == 1){
echo "« Previous";
}else{
$prevpage = $picid-1;
echo " <a href='".$_SERVER['PHP_SELF']."?viewpic=".$prevpage."&aid=".$album_id."'>« Previous</a> ";
}
echo " | ";
if($picid == $last_page){
echo "Next »";
}else{
$nextpage = $picid+1;
echo " <a href='".$_SERVER['PHP_SELF']."?viewpic=".$nextpage."&aid=".$album_id."'>Next »</a> ";
}
// Pagination end
echo "<br /><br />";
// Let's display full image
echo "<img src='".$image."' />";
}
}
// Now let's get all info that we need
$act = addslashes(htmlentities(htmlspecialchars($_REQUEST['act'])));
$view = addslashes(htmlentities(htmlspecialchars($_REQUEST['view'])));
$do = addslashes(htmlentities(htmlspecialchars($_REQUEST['do'])));
$pic = addslashes(htmlentities(htmlspecialchars($_REQUEST['viewpic'])));
// And here it starts to work
if($act != "" && $act == "new"){
if($do != "" && $do == "create"){
album::create_new_album();
}else{
album::create_new_album_form();
}
}elseif($act != "" && $act == "upload"){
if($do != "" && $do == "add"){
album::upload_image();
}else{
album::upload_image_form();
}
}elseif($act != "" && $act == "view"){
$id = addslashes(htmlentities(htmlspecialchars($_REQUEST['id'])));
if($id != "" && is_numeric($id)){
album::view_album();
}else{
break;
}
}elseif($pic != "" && is_numeric($pic)){
album::view_picture();
}else{
echo "<a href='index.php?act=new'>Create new album</a><br /><br />";
echo "<b>Albums:</b><br />";
album::list_albums();
}
?>
Code: Select all
How it works<?php
include("config.php");
$id = addslashes(htmlentities(htmlspecialchars($_REQUEST['id'])));
if(!is_numeric($id)){
die("Wrong ID! ID must be as a number.");
}
$sql = "SELECT * FROM images WHERE pic_id='".mysql_real_escape_string($id)."'";
$query = mysql_query($sql);
if(!$query){
die("Wrong ID");
}
$row = mysql_fetch_array($query);
$file = "http://forum.codecall.net/images/".$row['name'];
$file_ext_exp = explode(".", $file);
$file_ext = $file_ext_exp['1'];
$size = 0.10;
if($file_ext == "png"){
header('Content-type: image/png');
}elseif($file_ext == "jpg"){
header('Content-type: image/jpeg');
}elseif($file_ext == "gif"){
header('Content-type: image/gif');
}
list($width, $height) = getimagesize($file);
$thumbwidth = $width * $size;
$thumbheight = $height * $size;
$tn = imagecreatetruecolor($thumbwidth, $thumbheight);
if($file_ext == "png"){
$image = imagecreatefrompng($file);
}elseif($file_ext == "jpg"){
$image = imagecreatefromjpeg($file);
}elseif($file_ext == "gif"){
$image = imagecreatefromgif($file);
}
imagecopyresampled($tn, $image, 0, 0, 0, 0, $thumbwidth, $thumbheight, $width, $height);
if($file_ext == "png"){
imagepng($tn, null, 100);
}elseif($file_ext == "jpg"){
imagejpeg($tn, null, 100);
}elseif($file_ext == "gif"){
imagegif($tn, null, 100);
}
?>
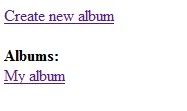
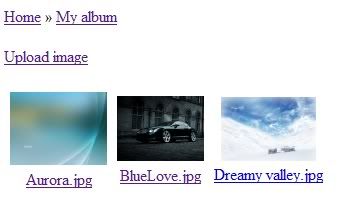
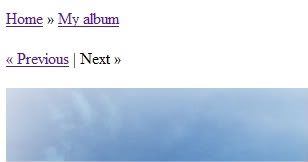
I hope you like it
And I hope it helped someone
Back Aegean sorry not been on i.v just been moving.
1 post
Page 1 of 1
Copyright Information
Copyright © Codenstuff.com 2020 - 2023